Sending Webhooks in Python
Dec 10, 2022Webhooks are a powerful tool for automating tasks and integrating applications. In a nutshell, a webhook is a way for one application to provide real-time information to another application by making a HTTP request to a specified URL. In this article, we'll look at how to send webhooks in Python.
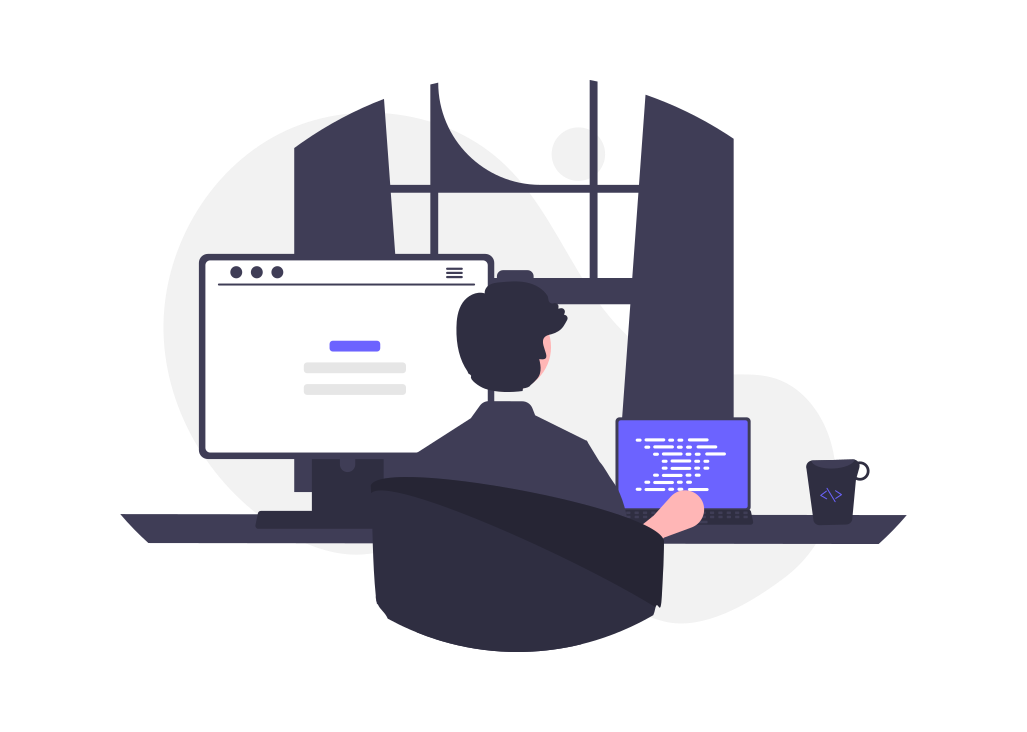
First, let's define a few terms that are commonly used when discussing webhooks:
- The sender is the application that sends the webhook.
- The receiver is the application that receives the webhook.
- The event is the action that triggers the webhook. For example, when a user signs up for a service, an event is triggered and a webhook is sent.
To send a webhook in Python, we'll need to use the
requests
pip install requests
Once you have the
requests
import requests webhook_url = 'https://www.example.com/webhook' data = { 'event': 'user_signed_up', 'user_id': 12345 } requests.post(webhook_url, json=data)
In the code above, we first import the
requests
data
user_signed_up
user_id
Finally, we use the
requests.post()
That's all there is to it! With just a few lines of code, you can easily send webhooks in Python. This can be a powerful tool for integrating applications and automating tasks.