Sending Webhooks in Go
Dec 10, 2022Webhooks are a powerful tool for automating tasks and integrating applications. In a nutshell, a webhook is a way for one application to provide real-time information to another application by making a HTTP request to a specified URL. In this article, we'll look at how to send webhooks in Go.
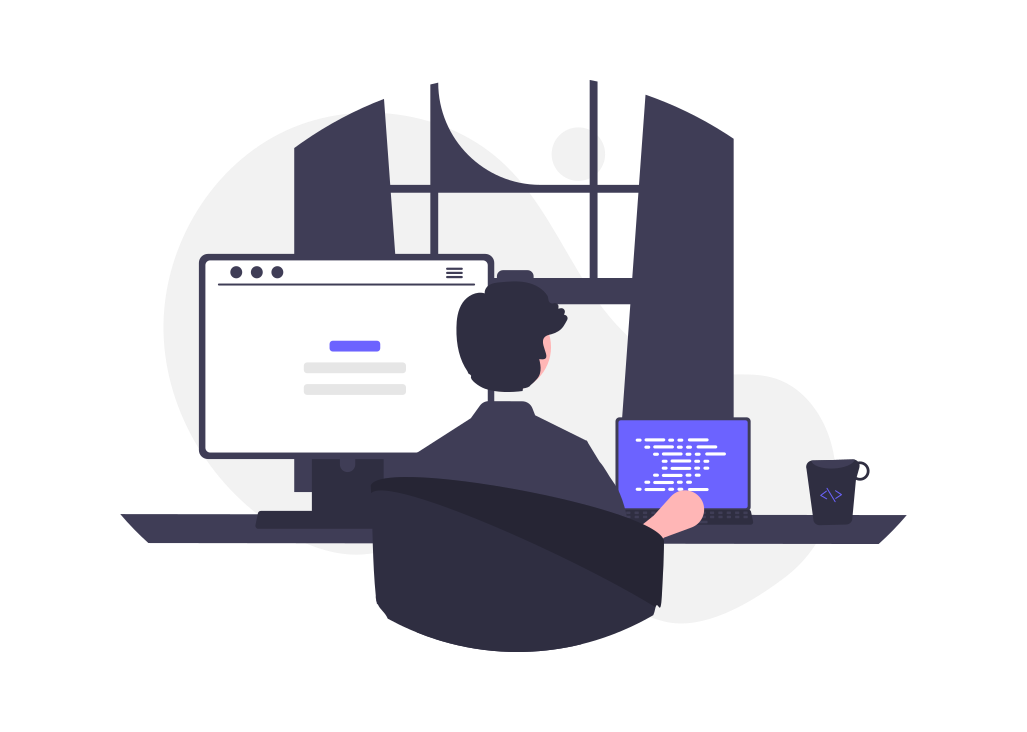
First, let's define a few terms that are commonly used when discussing webhooks:
- The sender is the application that sends the webhook.
- The receiver is the application that receives the webhook.
- The event is the action that triggers the webhook. For example, when a user signs up for a service, an event is triggered and a webhook is sent.
How to send a webhook in go
To send a webhook in Go, we'll need to use the
net/http
Here is an example of how to send a webhook in Go:
package main import ( "bytes" "encoding/json" "net/http" ) type WebhookData struct { Event string `json:"event"` UserID int `json:"user_id"` } func main() { webhookURL := "https://www.example.com/webhook" data := WebhookData{ Event: "user_signed_up", UserID: 12345, } jsonData, err := json.Marshal(data) if err != nil { log.error("unexpected error %v", err) } resp, err := http.Post(webhookURL, "application/json", bytes.NewBuffer(jsonData)) if err != nil { log.error("unexpected error %v", err) } defer resp.Body.Close() }
In the code above, we first define a
WebhookData
Event
UserID
Next, we define the main function where the webhook will be sent. We first define the URL of the webhook receiver as a variable called
webhookURL
We then create an instance of the
WebhookData
user_signed_up
user_id
Next, we use the
json.Marshal()
WebhookData
Finally, we use the
http.Post()
Content-Type
That's all there is to it! With just a few lines of code, you can easily send webhooks in Go. This can be a powerful tool for integrating applications and automating tasks.
Scaling your webhooks in Go
Scaling webhook delivery in your Go application is essential for ensuring robust and efficient communication between services. As your application grows, you will likely encounter challenges related to performance and reliability. Here are some strategies to effectively scale your webhooks and handle potential issues:
-
Queueing Mechanism: Implement an in-memory queue to manage outgoing webhooks. This queue will temporarily store webhook requests and process them in an orderly fashion, enhancing your application's throughput and reducing the risk of overloading your server or the recipient's server.
-
Rate Limiting and Batch Processing: Depending on the capabilities of the receiving server, consider implementing rate limiting or batch processing. Sending too many requests in a short time can overwhelm the recipient, leading to failed deliveries. By controlling the rate at which webhooks are sent or by batching multiple events into a single webhook, you can improve efficiency and reduce server load.
-
Retry Logic: Implement a retry mechanism for failed webhook deliveries. Network issues, server downtime, or other intermittent problems can cause webhook delivery failures. A retry mechanism with exponential backoff and jitter can help ensure successful delivery without overwhelming the server with immediate, repeated attempts.
-
Monitoring and Logging: It's crucial to monitor the performance of your webhook system. Implement logging for both successful and failed delivery attempts. This data can be invaluable for diagnosing issues, understanding the load on your system, and making informed decisions about scaling.
-
Using WebhookWizard: Consider integrating with WebhookWizard, a specialized service for managing webhooks. WebhookWizard can handle the complexities of queuing, rate limiting, and retry logic for you. By offloading these tasks, you can focus on your core application logic and ensure high reliability and performance of your webhook system.
Here's an example of how to implement an in-memory queue in Go with a limit on the number of items it can hold. This simple queue structure ensures that only a fixed number of webhook requests are held at any given time, preventing memory overflow:
package main
import (
"fmt"
"sync"
)
type WebhookQueue struct {
queue []WebhookData
limit int
mu sync.Mutex
}
func NewWebhookQueue(limit int) *WebhookQueue {
return &WebhookQueue{
queue: make([]WebhookData, 0),
limit: limit,
}
}
func (wq *WebhookQueue) Add(webhook WebhookData) error {
wq.mu.Lock()
defer wq.mu.Unlock()
if len(wq.queue) >= wq.limit {
return fmt.Errorf("queue limit reached")
}
wq.queue = append(wq.queue, webhook)
return nil
}
func (wq *WebhookQueue) Process() {
// Process the queue items
}
// WebhookData and other necessary code goes here
func main() {
queue := NewWebhookQueue(10) // Limit the queue to 10 items
// Example of adding a webhook to the queue
webhookData := WebhookData{Event: "user_signed_up", UserID: "12345"}
if err := queue.Add(webhookData); err != nil {
fmt.Println("Error adding to queue:", err)
}
// Process the queue (can be in a separate goroutine)
queue.Process()
}
In this example, the
WebhookQueue
Add
Process
Remember to continuously assess and adjust your scaling strategies as your application evolves. Integrating with Webhook Wizard can significantly simplify this process, allowing you to scale efficiently while maintaining high performance and reliability.